Table of Contents
How do you write if not in C++?
NOT: The NOT operator accepts one input. If that input is TRUE, it returns FALSE, and if that input is FALSE, it returns TRUE. For example, NOT (1) evaluates to 0, and NOT (0) evaluates to 1. NOT (any number but zero) evaluates to 0.
What does != Mean in C++?
not-equal-to operator
The not-equal-to operator ( != ) returns true if the operands don’t have the same value; otherwise, it returns false .

IS NOT statement in C?
The logical NOT operator is represented as the ‘!’ symbol, which is used to reverse the result of any given expression or condition. If the result of an expression is non-zero or true, the result will be reversed as zero or false value.
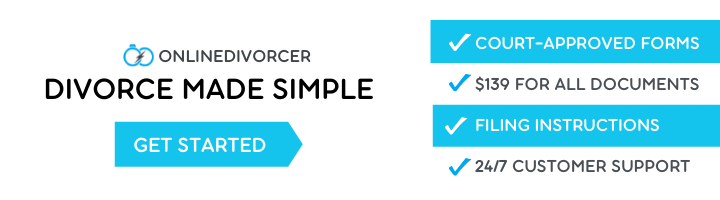
Can we use if without else in C++?
Yes, It is possible. You can’t write else without an if condition. The if condition checks whether your statement is true or false. If the statement is true the “if” condition will be executed else the program will stop running (if you had not provided the else condition).
How do you start writing an if statement in C++?
C++ has the following conditional statements:
- Use if to specify a block of code to be executed, if a specified condition is true.
- Use else to specify a block of code to be executed, if the same condition is false.
- Use else if to specify a new condition to test, if the first condition is false.
How does if statement work in C++?
C++ if Statement The if statement evaluates the condition inside the parentheses ( ) . If the condition evaluates to true , the code inside the body of if is executed. If the condition evaluates to false , the code inside the body of if is skipped.
Why is semicolon used in C++?
Role of Semicolon in C++: The Semicolon lets the compiler know that it’s reached the end of a command. Semicolon is often used to delimit one bit of C++ source code, indicating it’s intentionally separated from the respective code.
How do you write an if statement in C?
The example of an if-else-if statement in C language is given below.
- #include
- int main(){
- int number=0;
- printf(“enter a number:”);
- scanf(“%d”,&number);
- if(number==10){
- printf(“number is equals to 10”);
- }
What is else if statement in C?
else-if statements in C is like another if condition, it’s used in a program when if statement having multiple decisions. The basic format of else if statement is: if(test_expression) { //execute your code } else if(test_expression n) { //execute your code } else { //execute your code }
Are else statements necessary?
No, It’s not required to write the else part for the if statement. In fact most of the developers prefer and recommend to avoid the else block.
Can you have an if statement inside an if statement?
Yes, both C and C++ allow us to nested if statements within if statements, i.e, we can place an if statement inside another if statement.
What is an IF statement in C?
C – if statement. An if statement consists of a Boolean expression followed by one or more statements. Syntax. If the Boolean expression evaluates to true, then the block of code inside the ‘if’ statement will be executed.
What is the difference between and and not in C?
In C and C++ NOT is written as !. NOT is evaluated prior to both AND and OR. AND: This is another important command. AND returns TRUE if both inputs are TRUE (if ‘this’ AND ‘that’ are true).
What would happen if there was no conditional statement in C?
Without a conditional statement such as the if statement, programs would run almost the exact same way every time. If statements allow the flow of the program to be changed, and so they allow algorithms and more interesting code.
What is the difference between if and else in C++?
if..else statements In an if…else statement, if the code in the parenthesis of the if statement is true, the code inside its brackets is executed. But if the statement inside the parenthesis is false, all the code within the else statement’s brackets is executed instead.