Table of Contents
Is recursion used in linked list?
Most recursive methods operating on linked list have a base case of an empty list; most have a recursive call on the next instance variable, which refers to a smaller list: one that contains one fewer node.
How do you create a recursive linked list?
Allocate the new Node in the Heap using malloc() & set its data. Recursively set the next pointer of the new Node by recurring for the remaining nodes. Return the head pointer of the duplicate node. Finally, print both the original linked list and the duplicate linked list.

How nodes can be inserted in a linked list recursively?
You call the function. head is not equal to NULL. So you need to call the function recursively for head->next and then set the data member head->next with the pointer of the newly created node returned from the recursive call of the function.
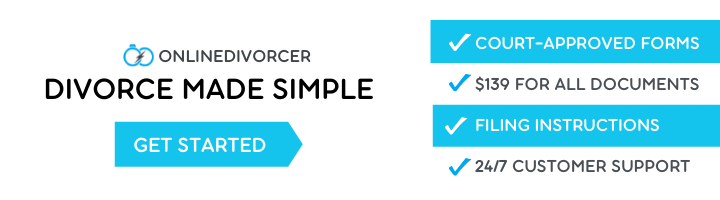
What is recursion in C++?
Recursion is a method in C++ which calls itself directly or indirectly until a suitable condition is met. In this method, we repeatedly call the function within the same function, and it has a base case and a recursive condition.
What is meant by recursion?
Definition of recursion 1 : return sense 1. 2 : the determination of a succession of elements (such as numbers or functions) by operation on one or more preceding elements according to a rule or formula involving a finite number of steps.
What is recursion example?
Recursion is the process of defining a problem (or the solution to a problem) in terms of (a simpler version of) itself. For example, we can define the operation “find your way home” as: If you are at home, stop moving. Take one step toward home.
How do you do recursion in C++?
C++ Recursion Example
- #include
- using namespace std;
- int main()
- {
- int factorial(int);
- int fact,value;
- cout<<“Enter any number: “;
- cin>>value;
What is recursion Easter?
It is like two mirrors facing each other and displaying an infinite trail of opposite images. In recursion, the objects are repeated infinite times. The iteration runs forever. Google displays this quality when you search for recursion.
What is recursion in C++? Give example?
The process in which a function calls itself is known as recursion and the corresponding function is called the recursive function. The popular example to understand the recursion is factorial function. Factorial function: f(n) = n*f(n-1), base condition: if n<=1 then f(n) = 1.
What is recursion trick in Google?
Go to google.com and search “recursion”. Normal results will appear. Now all you need to do is press the “did you mean recursion” and you will be stuck in a loop. Watch the homepage come crashing down with the google gravity trick.
How to create a linked list?
The first node,head,will be null when the linked list is instantiated.
How to display elements in a linked list by recursion?
– Create and build the linked list – Display the original linked list – Display in reverse order
What is an example of a linked list?
Singly Linked List. Singly linked lists contain nodes which have a data field as well as a next field,which points to the next node in the sequence.
What is a simple linked list?
Simple Linked List − Item navigation is forward only.